Python script for Windows
import os
def calculate_folder_details(folder_path):
"""Calculate the size of a folder in bytes and count the number of files."""
total_size = 0
file_count = 0
for dirpath, dirnames, filenames in os.walk(folder_path):
file_count += len(filenames)
for file in filenames:
filepath = os.path.join(dirpath, file)
if os.path.exists(filepath):
total_size += os.path.getsize(filepath)
return total_size, file_count
def format_size(size_in_bytes):
"""Convert size from bytes to GB and MB."""
size_in_gb = size_in_bytes / (1024 ** 3) # Convert bytes to GB
size_in_mb = size_in_bytes / (1024 ** 2) # Convert bytes to MB
return size_in_gb, size_in_mb
def main():
base_path = 'D:\\_FTP_install_home\\' # Replace 'YourUsername' with the actual username
output_file = 'D:\\espacio-GB-2025-01-23.txt' # Replace 'YourUsername' with the actual username
# Ensure base path exists
if not os.path.exists(base_path):
print(f"Error: The path {base_path} does not exist.")
return
folder_details = []
total_size_bytes = 0
total_files = 0
for item in os.listdir(base_path):
item_path = os.path.join(base_path, item)
if os.path.isdir(item_path):
folder_size_bytes, file_count = calculate_folder_details(item_path)
size_in_gb, size_in_mb = format_size(folder_size_bytes)
total_size_bytes += folder_size_bytes
total_files += file_count
folder_details.append((item, size_in_gb, size_in_mb, file_count))
# Sort by size in GB (descending)
folder_details.sort(key=lambda x: x[1], reverse=True)
with open(output_file, 'w') as f:
# Column headers with left alignment
f.write(f'{"Folder":<30}{"Size (GB)":<15}{"Size (MB)":<15}{"File Count":<10}\n')
for item, size_in_gb, size_in_mb, file_count in folder_details:
f.write(f'{item:<30}{size_in_gb:<15.2f}{size_in_mb:<15.2f}{file_count:<10}\n')
print(f"Folder details written to {output_file}")
if __name__ == "__main__":
main()
Python script for Linux
import os
def calculate_folder_details(folder_path):
"""Calculate the size of a folder in bytes and count the number of files."""
total_size = 0
file_count = 0
for dirpath, dirnames, filenames in os.walk(folder_path):
file_count += len(filenames)
for file in filenames:
filepath = os.path.join(dirpath, file)
if os.path.exists(filepath):
total_size += os.path.getsize(filepath)
return total_size, file_count
def format_size(size_in_bytes):
"""Convert size from bytes to GB and MB."""
size_in_gb = size_in_bytes / (1024 ** 3) # Convert bytes to GB
size_in_mb = size_in_bytes / (1024 ** 2) # Convert bytes to MB
return size_in_gb, size_in_mb
def main():
base_path = '/LUSTRE/home/jant.encinar.umh.es/' # Linux path, replace with the correct one
output_file = '/LUSTRE/home/jant.encinar.umh.es/espacio-home-2025-01-23.txt' # Output file path in Linux
# Ensure the base path exists
if not os.path.exists(base_path):
print(f"Error: The path {base_path} does not exist.")
return
folder_details = []
total_size_bytes = 0
total_files = 0
# Iterate over the items in the base path
for item in os.listdir(base_path):
item_path = os.path.join(base_path, item)
if os.path.isdir(item_path): # Only directories
folder_size_bytes, file_count = calculate_folder_details(item_path)
size_in_gb, size_in_mb = format_size(folder_size_bytes)
total_size_bytes += folder_size_bytes
total_files += file_count
folder_details.append((item, size_in_gb, size_in_mb, file_count))
# Sort by size in GB (descending)
folder_details.sort(key=lambda x: x[1], reverse=True)
# Save folder details to the output file
with open(output_file, 'w') as f:
f.write(f'{"Folder":<30}{"Size (GB)":<15}{"Size (MB)":<15}{"File Count":<10}\n')
for item, size_in_gb, size_in_mb, file_count in folder_details:
f.write(f'{item:<30}{size_in_gb:<15.2f}{size_in_mb:<15.2f}{file_count:<10}\n')
print(f"Folder details written to {output_file}")
if __name__ == "__main__":
main()
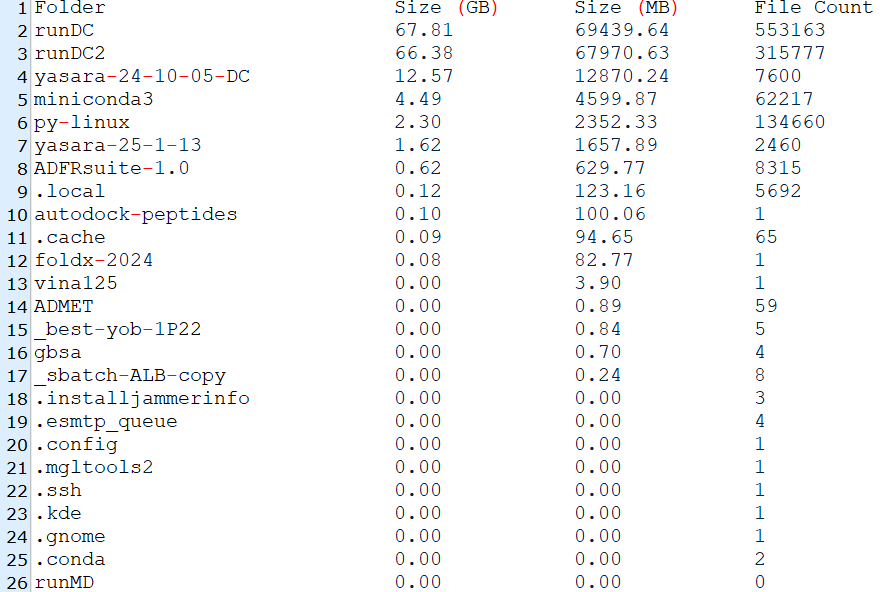